Stopping node.js – an elegant way
If you want to stop node.js you have basically two ways:
– brute force (β – C),
– gentle way, via socket inside your application.
I will show you how to do that using simple code (https://nodejs.org/en/about/).
/* app.js */ const http = require('http'); const hostname = '127.0.0.1'; const port = 3000; const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World\n'); }); server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`); });
Brute force
This is the simplest way, just kill your app inside console using Ctrl-C (macOS: ^-C). All we have to do is to start node (node app.js) and terminate it.
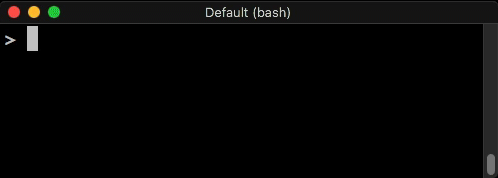
Gentle way
Here, we are using socket to listen for messages from client. We have to modify server a little bit, we have to add socket.
/* app.js */ const http = require('http'); const hostname = '127.0.0.1'; const port = 3000; const server = http.createServer((req, res) => { res.statusCode = 200; res.setHeader('Content-Type', 'text/plain'); res.end('Hello World\n'); }); server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`); }); const io = require('socket.io')(server); io.on('connection', (socketServer) => { socketServer.on('stop', () => { process.exit(0); }); });
Make sure to install socket packages as well
> npm install socket.io > npm install socket.io-client
Once we have server part, we can focus on client
/* app.stop.js */ const io = require('socket.io-client'); const socketClient = io.connect('http://127.0.0.1:3000/'); socketClient.on('connect', () => { socketClient.emit('stop'); setTimeout(() => { process.exit(0); }, 1000); });
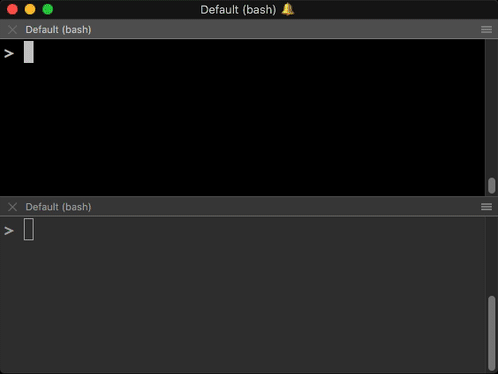