You need no maven to run JUnit 5 tests
Whenever I see presentation, article, tutorial, related to JUnit 5 based tests there is Maven as well. I am not quite a fan of Maven. Anyway, I think it’s worth remembering that for running tests you don’t need it. You don’t have to create complex pom.xml file. You can resort to just few lines of code. All you have to do, is to use make.
Let’s say I have following structure of directory
. |-- Makefile `-- src |-- main | `-- sample | `-- Main.java `-- test `-- sample `-- MainTest.java
and my files look like this
package sample; public class Main { public int add(int a, int b) { return a + b; } }
I want to run test that will make sure it’s valid. I can prepare something like this
package sample; import static org.junit.jupiter.api.Assertions.assertEquals; import org.junit.jupiter.api.DisplayName; import org.junit.jupiter.api.Test; class MainTest { @Test @DisplayName("2 + 2 = 4") void twoPlusTwoEqualsFour() { Main m = new Main(); assertEquals(4, m.add(2,2), "Something went wrong!"); } }
I will also need very simple Makefile
assert = $(if $2,$(if $1,,$(error $2))) JUnit.ver = 1.5.2 JUnit.jar = junit-platform-console-standalone-$(JUnit.ver).jar Maven.http = http://central.maven.org/maven2/org/junit/platform/junit-platform-console-standalone JUnit.mvn = $(Maven.http)/$(JUnit.ver)/$(JUnit.jar) all: check-variable test check-variable: $(call assert,$(JAVA_HOME),JAVA_HOME is not defined) dirs: -mkdir -p target -mkdir -p lib compile-java: dirs $(JAVA_HOME)/bin/javac -d target/classes src/main/java/sample/*.java junit-download: curl -s -z lib/$(JUnit.jar) \ -o lib/$(JUnit.jar) \ $(JUnit.mvn) compile-test: compile-java junit-download $(JAVA_HOME)/bin/javac -d target/test-classes \ -cp lib/$(JUnit.jar):target/classes \ src/test/java/sample/*.java test: compile-test $(JAVA_HOME)/bin/java -jar lib/$(JUnit.jar) \ --class-path target/classes:target/test-classes \ --scan-class-path clean: -rm -rf lib -rm -rf target
and then, I can simply run make
> make ... ... Thanks for using JUnit! Support its development at https://junit.org/sponsoring ╷ ├─ JUnit Jupiter ✔ │ └─ MainTest ✔ │ └─ 2 + 2 = 4 ✔ └─ JUnit Vintage ✔ Test run finished after 153 ms [ 3 containers found ] [ 0 containers skipped ] [ 3 containers started ] [ 0 containers aborted ] [ 3 containers successful ] [ 0 containers failed ] [ 1 tests found ] [ 0 tests skipped ] [ 1 tests started ] [ 0 tests aborted ] [ 1 tests successful ] [ 0 tests failed ]
You can download sample code here
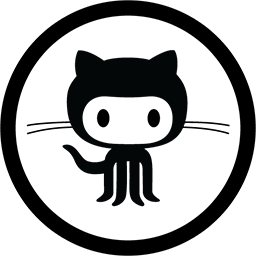